Static Maps API
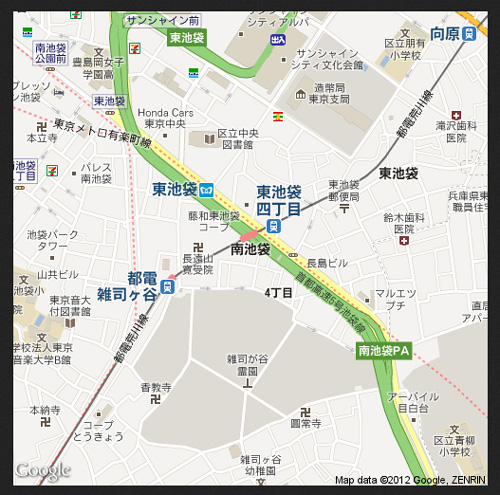
<html>
<head>
<meta charset="utf-8">
<title>Google Map Test</title>
</head>
<body>
<a href="http://maps.google.com/maps/api/staticmap?center=35.724943,139.719913&zoom=16&size=500x500&maptype=roadmap&sensor=false">マップで見る</a>
</body>
</html>

<html>
<head>
<meta charset="utf-8">
<title>Google Map API</title>
<script type="text/javascript" src="http://maps.google.com/maps/api/js?sensor=true&language=ja"></script>
<script>
function initialize() {
var latlng = new google.maps.LatLng(35.724943, 139.719913);
var myOptions = {
zoom: 13,
center: latlng,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
var map = new
google.maps.Map(document.getElementById("map_canvas"),myOptions);
var marker1 = new google.maps.Marker({
position: latlng,
title:"南池袋",
});
marker1.setMap(map);
var myLatlng = new google.maps.LatLng(35.718278,139.705824);
var marker2 = new google.maps.Marker({
position: myLatlng,
title:"切手の博物館"
});
marker2.setMap(map);
var marker1text = 'ここは<span style="color:#0066cc;">南池袋</span>です'
var infowindow1 = new google.maps.InfoWindow({
content: marker1text,
maxWidth: 200
});
google.maps.event.addListener(marker1, 'click', function() {
infowindow1.open(map,marker1);
});
var marker2text = 'ここは<span style="color:#0066cc;">切手の博物館</span>です'
var infowindow2 = new google.maps.InfoWindow({
content: marker2text,
maxWidth:200
});
google.maps.event.addListener(marker2, 'click', function() {
infowindow2.open(map, marker2);
})
}
</script>
</head>
<body onLoad="initialize()">
<div id="map_canvas" style="width: 640px; height: 480px;"></div>
</body>
</html>
ルート検索マップ(詳細)
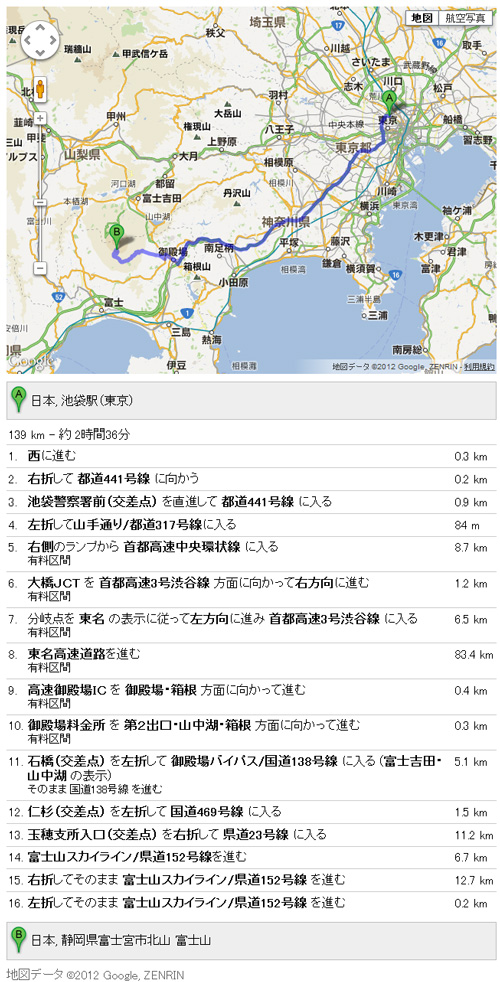
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>Google Maps API SDK</title>
<script type="text/javascript" src="http://maps.google.com/maps/api/js?sensor=true&language=ja"></script>
<script type="text/javascript">
function initialize(position) {
var latlng = new google.maps.LatLng(35.728926,139.71038);
var myOptions = {
zoom: 15,
center: latlng,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
var rendererOptions =
{
draggable: true,
preserveViewport:false
};
var directionsDisplay = new google.maps.DirectionsRenderer(rendererOptions);
var directionsService = new google.maps.DirectionsService();
var request =
{
origin: "池袋",
destination: "富士山",
travelMode: google.maps.DirectionsTravelMode.DRIVING,
unitSystem: google.maps.DirectionsUnitSystem.METRIC,
optimizeWaypoints: true,
avoidHighways: false,
avoidTolls: false
};
directionsService.route(request, function(response, status)
{
if (status == google.maps.DirectionsStatus.OK)
{
directionsDisplay.setDirections(response);
directionsDisplay.setPanel(document.getElementById("directionsPanel"));
}
});
var map = new google.maps.Map(document.getElementById("map_canvas"), myOptions);
directionsDisplay.setMap(map);
}
</script>
</head>
<body onload="initialize()">
<div id="map_canvas" style="width: 640px; height: 480px;"></div>
<div id="directionsPanel" style="width: 640px; height: 480px;"></div>
</body>
</html>
池袋から学校までのルートマップ

<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="initial-scale=1.0, user-scalable=no">
<title>ルートマップ</title>
<style>
html, body {
margin: 0;
padding: 0;
}
div#map_canvas {
width: 500px;
height: 500px;
}
</style>
<script src="http://maps.google.com/maps/api/js?sensor=true"></script>
<script>
var map;
var directionsDisplay = new google.maps.DirectionsRenderer;
var directionsService = new google.maps.DirectionsService();
function initialize() {
var myOptions = {
center:new google.maps.LatLng(35.728926,139.71038),
zoom: 13,
mapTypeId:google.maps.MapTypeId.ROADMAP,
};
map = new google.maps.Map(document.getElementById("map_canvas"), myOptions);
directionsDisplay.setMap(map);
calcRoute();
}
var end = new google.maps.LatLng(35.724442,139.715447);
function calcRoute() {
var request = {
origin: "池袋",
destination:end,
travelMode: google.maps.DirectionsTravelMode.DRIVING,
optimizeWaypoints: true,
};
directionsService.route(request, function(response, status) {
if (status == google.maps.DirectionsStatus.OK) {
directionsDisplay.setDirections(response);
}
});
}
</script>
</head>
<body onload="initialize();">
<div id="map_canvas" style="width: 500px; height: 500px;"></div>
</body>
</html>